Monitoring Progress of a Calculation
Users sometimes want to monitor the progress of some calculation or simulation. Here's a recent example from the MATLAB newsgroup.
Contents
Some functions have an option you can set that displays information throughout the course of a calculation, for example, fminsearch via the OutputFcn in optimset. What are your choices when you want some intermediate output from your own code? I see three choices.
- Mimic behavior of the OutputFcn idea in your own code so you can specify the output.
- Use waitbar for a graphic progress meter.
- Send output to the command window in a way that's legible and doesn't clutter the information, using fprintf and taking advantage of some of the special formatting capabilities.
Graphical Output Option Using waitbar
Here's a very short illustration of the kind of control you can get using waitbar. First, create a waitbar and get its handle.
h = waitbar(0,'Initializing waitbar...');
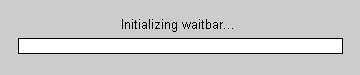
Here's an update when the calculation is halfway done. Make sure to use the waitbar handle to update the existing waitbar.
waitbar(0.5,h,'Halfway there...')

And further along again:
perc = 75;
waitbar(perc/100,h,sprintf('%d%% along...',perc))

Close the progess meter when the calculation is done.
close(h)
There are a plethora of alternatives to waitbar on The MATLAB Central File Exchange. I count 29 today when I search for waitbar.
Textual Output Option
fprintf can print output to the command window by specifying the file identifier as the value 1. In addition, you can take advantage of escape characters, such as \b for backspace, to control where the output goes.
Look at lines 2 and 4 in the code snippet here. On line 2, I print out a line, but do not terminate it with a newline character \n. On line 4, we use the backspace capability to overwrite our previous integer, before pausing so the command window output can be updated. Finally, when the loop is complete, I use fprintf to advance the cursor to a new line \n.
dbtype cmdwinProgress
clc
1 % Example Code for Printing Progress to the Command Window 2 fprintf(1,'here''s my integer: '); 3 for i=1:9 4 fprintf(1,'\b%d',i); pause(.1) 5 end 6 fprintf('\n')
Here's the final result from that command window, having first made sure the screen (using clc) before running cmdwinProgress.
Progress Indicators
What do you look for in a progress indicator? Do any of the options mentioned above work for you? If not, why not? Post your thoughts here.
- Category:
- Less Used Functionality